Admob for MAUI made easy
In this tutorial I’ll show you how to monetize your MAUI apps with AdMob using my MauiMTAdmob plugin.
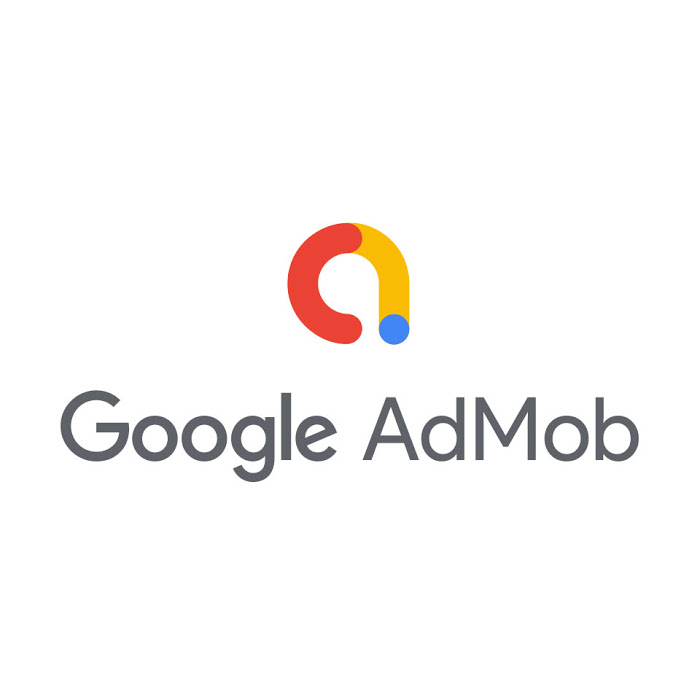
To help you to speed up your MAUI development, I’ve created a set of plugins, one of them is MauiMTAdmob. Thanks to this plugin you can add Admob banners, Insterstitials, and Rewarded ads in just few lines of code. It couldn’t be easier than that and I’ll show you.
Install the plugin
First of all, right click on your MAUI solution and select “Manage Nuget packages for Solution”. Visual Studio will open a new screen where you can search and install one or more nuget packages. In this case we can search for the MauiMTAdmob plugin and select it as showed in the next image.
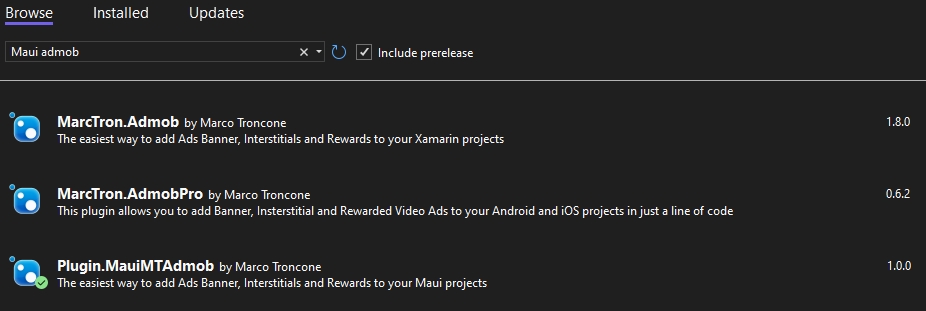
After the Admob plugin is installed we can add banners, interstitials, rewarded and rewarded interstitials to our projects.
IMPORTANT FOR ANDROID
If you are receiving some errors about UMP while compiling your projects, add the following code to your csproj file:
<ItemGroup Condition="'$(TargetFramework)' == 'net6.0-android'">
<AndroidLibrary Include="Dependencies\user-messaging-platform-2.0.0.aar" Bind="false" />
</ItemGroup>
Now, follow these steps:
- Create a folder “Dependencies” in your project
- Download the file user-messaging-platform-2.0.0.aar from internet or from the MauiMTAdmob sample repository (https://github.com/marcojak/MauiMTAdmob)).
- Copy the file in that folder
- Build your project and everything will compile correctly
Add Ads to our project
MauiMTAdmob plugin supports banner, interstitials and rewarded videos for Android and iOS. If you would like to see the plugin supporting also other platforms let me now and I’ll try to add the support in a new version.
As I’ve said we can add Banners, Interstitials and Rewarded Videos ads to our project. Let’s start with the Banners
How to add an Admob Banner
An Admob banner is just a view inside our page. It means that we can add it using XAML or C#. First of all let’s see how to add an Admob banner using XAML.
Add an Admob Banner with XAML
In MauiMTAdmob to use an Admob banner I’ve created a custom control called MTAdView, so to use it we can use this code:
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:controls="clr-namespace:Plugin.MauiMTAdmob.Controls;assembly=Plugin.MauiMTAdmob"
x:Class="SampleMMTAdmob.MainPage">
<StackLayout>
<Label Text="Let's test an Admob Banner!"
HorizontalOptions="Center"
VerticalOptions="CenterAndExpand" />
<!-- Place the Admob controls here -->
<controls:MTAdView/>
</StackLayout>
In this example we have created a StackLayout with 2 controls: a label and an AdView (our Admob banner). Easy! Isn’t it???
The AdView control is basically a View so you can use all the properties you can think of like: HorizontalOptions, VerticalOptions, IsVisible…
In addition to these properties, I’ve added in AdView two other properties: AdsId and PersonalizedAds.
AdsId: Allows you to add the Banner Id (you can find it in your Admob account)
PersonalizedAds: This allow you to use non personalized ads. For example in case of GPDR. Of course it’s better to use personalized Ads, but please test your ads as I cannot guarantee it works as intended.
To use these properties you can update the previous code to:
<controls:AdView PersonalizedAds="true" AdsId="xxxxxxxxxxxxxxxxxx"/>
Add an Admob Banner with C#
In case you don’t write your pages with XAML or you write your UI in C# or you want to add your view only in some cases, you can add your Admob Banner using this code:
using Plugin.MauiMTAdmob;
...
MTAdView ads = new MTAdView();
Of course you need to attach this View to your layout, but you know how to do it (If not, feel free to ask).
To use the custom properties you can change the previous code to:
...
MTAdView ads = new MTAdView();
ads.AdsId = "xxx";
ads.PersonalizedAds = true;
Also in this case, to add an Admob banner is INCREDIBILY EASY!!!
Global Custom Properties
As you have seen, the properties AdsId and PersonalizedAds belong to a single AdView. It means that you have to set them for every Admob Banner.
To make things even easier I’ve added the option to set these properties only once. To do so, you can use this C# code:
CrossMauiMTAdmob.Current.UserPersonalizedAds = true;
CrossMauiMTAdmob.Current.AdsId = "xxxxxxxxxxxxxxxx";
In this case all your Admob banner will show personalized ads and will have the same Id.
If you set local and global properties, the local ones will have higher priority.
Use of Banner Events
I’ve added 4 events to the Admob banner that you could find nice to have. These events are:
- AdsClicked When a user clicks on the ads
- AdsClosed When the user closes the ads
- AdsImpression Called when an impression is recorded for an ad.
- AdsOpened When the ads is opened
- AdsLoaded When the ads is loaded
- AdsFailedToLoad When the ads fails to load
To use these events you can write this code:
AdView myAds = new AdView();
myAds.AdsClicked += ...;
myAds.AdsClosed += ...;
myAds.AdsImpression += ...;
myAds.AdsOpened += ...;
...
Of course you can use these events also if you have declared your AdView in your XAML code.
Admob Interstitials
Now that we know how to add Admob banners using my plugin MauiMTAdmob , let’s see how we can add Admob Interstitials. If possible, to add an Admob interstitial is even easier. You just need a single line of code. Don’t you believe me? Look here how to show an Admob interstitial:
CrossMauiMTAdmob.Current.ShowInterstitial("ca-app-pub-xxxxxxxxxxxxxxxx/xxxxxxxxxx");
I told you!!! That’s it!!! With that line of code you have just showed an Interstitial in you app. Of course you need to replace that string with the Insterstitial ID you can find in your Admob account.
To load an Interstitial you can use:
CrossMauiMTAdmob.Current.LoadInterstitial(INTERSTITIALID);
and to check if an Interstitial is loaded:
CrossMauiMTAdmob.Current.IsInterstitialLoaded()
Events for Interstitials
There 3 events that you can use with Interstitials:
OnInterstitialLoaded
OnInterstitialOpened
OnInterstitialClosed
OnInterstitialFailedToLoad
OnInterstitialFailedToShow
OnInterstitialClicked
OnInterstitialImpression
Rewarded
To show a rewarded video you just need a single line of code:
CrossMTAdmob.Current.ShowRewardedVideo("xx-xxx-xxx-xxxxxxxxxxxxxxxxx/xxxxxxxxxx");
To load a Rewarded video:
CrossMTAdmob.Current.LoadRewarded("xx-xxx-xxx-xxxxxxxxxxxxxxxxx/xxxxxxxxxx");
To check if a Rewarded video is loaded:
CrossMTAdmob.Current.IsRewardedLoaded("xx-xxx-xxx-xxxxxxxxxxxxxxxxx/xxxxxxxxxx");
Events for Rewarded
There are 7 events that you can use with the Rewarded Ads:
OnRewardedLoaded
OnRewardedOpened
OnRewardedClosed
OnRewardedImpression
OnRewardedClicked
OnUserEarnedReward
Rewarded Interstitial
To show a rewarded video you just need a single line of code:
CrossMTAdmob.Current.ShowRewardInterstitial(“xx-xxx-xxx-xxxxxxxxxxxxxxxxx/xxxxxxxxxx”);
To load a Rewarded video:
CrossMTAdmob.Current.LoadRewardInterstitial(“xx-xxx-xxx-xxxxxxxxxxxxxxxxx/xxxxxxxxxx”);
To check if a Rewarded video is loaded:
CrossMTAdmob.Current.IsRewardInterstitialLoaded(“xx-xxx-xxx-xxxxxxxxxxxxxxxxx/xxxxxxxxxx”);
Events for Rewarded Interstitials
There are 7 events that you can use with the Rewarded Interstitials:
OnRewardedLoaded
OnRewardedOpened
OnRewardedClosed
OnRewardedImpression
OnRewardedClicked
OnUserEarnedReward
Initialization
Before you can use the Admob banners, Interstitials and Rewarded, you need to initialize it.
First of all in your CreateMauiApp() add this to your builder:
.UseMauiMTAdmob()
You need to do it only once so it makes sense to initialize it in the OnCreate method in Android and FinishedLaunching in iOS.
In your Android project add this line in your OnCreate method:
MobileAds.Initialize(this);
Remeber to add this to your AppManifest:
<!-- Sample AdMob App ID: ca-app-pub-3940256099942544~3347511713 -->
<meta-data android:name="com.google.android.gms.ads.APPLICATION_ID"
android:value="ca-app-pub-xxxxxxxxxxxxxxxx~yyyyyyyyyy"/>
In your iOS project add this line in your FinishedLaunching method:
MobileAds.SharedInstance.Start(CompletionHandler);
private void CompletionHandler(InitializationStatus status){}
On iOS you need to add to your Info.plist file this:
<key>GADApplicationIdentifier</key>
<string>YOUR APP ID</string>
<key>GADIsAdManagerApp</key>
<true/>
Some useful links
- Admob official website: https://apps.admob.com/
- Available on Nuget: https://www.nuget.org/packages/MarcTron.Admob
- Sample Source Code: https://github.com/marcojak/MauiMTAdmob
- Tutorial: https://www.mauiexpert.it/admob-for-maui-made-easy/
- To report any issue: https://github.com/marcojak/MauiMTAdmob/issues
Conclusion
This Admob MauiMTAdmob plugin is incredibly easy to use but in case you need help, or you want to suggest a new feature or for any other reason, write me.
Disclaimer
This plugin is free of charge and you may use it as you like, but regardless of your situation and usage, I won’t be held responsible of any loss.
It’s your responsibility to comply with every regulation around the word needed to show personalized and not personalized ads to the user.
Even though this plugin might support functionalities to help to comply with some regulations, it’s your responsibility to verify that everything works as intended and to implement any functionality that might be necessary.
21 December 2022 @ 5:11 PM
Ciao Marco, grazie per il tuo lavoro, Google chiede di dichiarare l’utilizzo l’eventuale utilizzo dell’ID Pubblicità, anche in caso di uso di librerie di terze parti, devo rispondere SI oppure No.
Ringrazio in anticipo per un tuo riscontro
25 November 2022 @ 3:53 AM
Thank you veyr much for this. Do you think this should work ok with dotnet7 too?
21 June 2023 @ 12:09 PM
I have the same question. I recently upgraded to net7.0 and have been recieving build errors:
“D:\.nuget\packages\xamarin.firebase.ios.core\8.10.0.3\lib\net6.0-ios15.4\Firebase.Core.resources\GoogleAPIClientForREST.xcframework\ios-arm64_x86_64-simulator\GoogleAPIClientForREST.framework\Headers\GoogleAPIClientForREST-umbrella.h” to “bin\Debug\net7.0-ios\iossimulator-x64\Firebase.Core.resources\GoogleAPIClientForREST.xcframework\ios-arm64_x86_64-simulator\GoogleAPIClientForREST.framework\Headers\GoogleAPIClientForREST-umbrella.h”. Exceeded retry count of 10. Failed. TrigonometryCalculatorV2 D:\Program Files\Microsoft Visual Studio\2022\MSBuild\Current\Bin\amd64\Microsoft.Common.CurrentVersion.targets 4862
10 November 2022 @ 8:50 PM
¡Muchas gracias marcojak!, por tu trabajo, se me presentaron varios inconvenientes que pude resolver y ya pude implementar admob en mi app,
10 November 2022 @ 8:04 PM
tengo este mismo problema
10 November 2022 @ 2:32 PM
Cual es la forma correcta de recudir esta ruta, se hace mediante el visual studio? , me refiero a donde puedo cambiar la ruta de la forma indicada
10 November 2022 @ 5:20 AM
excelente trabajo, he intentado instalarlo para MAUI pero también me sale el error de que la ruta está incompleta, Error Could not find a part of the path ‘C:\Users\DAX.nuget\packages\xamarin.firebase.ios.core\8.10.0.1\lib\net6.0-ios15.4\Firebase.Core.resources\GoogleUtilitiesComponents.xcframework\ios-arm64_i386_x86_64-simulator\GoogleUtilitiesComponents.framework\PrivateHeaders\GULCCComponentContainerInternal.h’.
Podrías explicar como se soluciona esto?
10 November 2022 @ 8:35 AM
Hi. The nuget path is too long. You need to reduce it! Eh. C:\nuget...
9 November 2022 @ 9:30 PM
Great work sir…… but when am try to download it am getting this…. error message
‘C:\Users\Samvev.nuget\packages\xamarin.firebase.ios.core\8.10.0.1\lib\net6.0-ios15.4\Firebase.Core.resources\GoogleUtilitiesComponents.xcframework\ios-arm64_i386_x86_64-simulator\GoogleUtilitiesComponents.framework\Headers\GoogleUtilitiesComponents-umbrella.h’.
9 November 2022 @ 10:31 PM
I think the reason is that your nuget path is too long. You need to reduce it.
21 October 2022 @ 1:25 AM
edit Platforms/Android/MainActivity.cs .
insert in MainActive:
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
MobileAds.Initialize(this);
}
19 October 2022 @ 5:39 PM
Hi i’m new to .NET Maui, but I’m stuck on the instructions above. It says in the initialization section:
“In your Android project add this line in your OnCreate method:
MobileAds.Initialize(this);”
But i don’t have android project as it’s crossplatform. Am i missing something? It would be ahelpful also to have a tutorial video to follow. Thanks
19 October 2022 @ 5:16 PM
Hi, I’ve followed all of the steps above to add a banner ad, but when running the project I’m getting an error:
“Java.Lang.RuntimeException: ‘Unable to get provider com.google.android.gms.ads.MobileAdsInitProvider”.
Any idea what the cause and solution are? Thanks
9 January 2023 @ 4:10 PM
Same problem, did you find a solution?
12 October 2022 @ 9:59 AM
I have used AdsJumbo in one of my UWP projects but monetization is not as good as with Admob. Another one I tried in an UWP was the Vungle SDK. Monetization was similar to AdsJumbo.
Advantage of Vungle is that they claim to have no depencies. A third one is AdDeals but I haven’t tried that in an app.
Unfortunately, at the time of this writing all the available nuget packages (Vungle SDk 6.11, AdDealsUnversalSDKW81 4.74, AdsJumbo 5.9.1) raise errors when you try to install them in a standard .NET MAUI project.
21 September 2022 @ 7:09 PM
Hi marcojak,
Congratulations for the great work. unfortunately I can not install the nuget package either, the path is too long.
I have searched the internet for a solution, but nothing works.
Is the path of the installation specified by you? It would be enough if the last directory was shortened a bit.
Here is the directory where Nuget tries to install package:
C:\Users\Sasa.nuget\packages\xamarin.firebase.ios.core\8.10.0.1\lib\net6.0-ios15.4\Firebase.Core.resources\GoogleUtilitiesComponents.xcframework\ios-arm64_i386_x86_64-simulator\GoogleUtilitiesComponents.framework\Headers
Thanks
sasa
21 September 2022 @ 8:38 PM
Hi Sasa, try to change the nuget folder to c:\nuget…it should work
5 September 2022 @ 2:36 PM
HI I am using Adsjumbo for my UWP apps (https://adsjumbo.com/) may be this is helpfull
Martin
5 September 2022 @ 3:25 PM
Hi Martin, this is interesting. I’ll see if I can integrate this plugin in my plugin… technically it shouldn’t be too difficult, but I want to find the best way to do it, so that potentially an user could chose his own preferred uwp plugin
3 September 2022 @ 3:46 PM
Plugin looks great, but I am getting an error message when trying install the NuGet and I am unsure what the cause is or how to resolve. Any help would be great. The message is: Could not find a part of the path ‘C:\Users\geral.nuget\packages\xamarin.firebase.ios.core\8.10.0.1\lib\xamarinios10\Firebase.Core.resources\GoogleUtilitiesComponents.xcframework\ios-arm64_i386_x86_64-simulator\GoogleUtilitiesComponents.framework\PrivateHeaders\GULCCComponentContainerInternal.h’
3 September 2022 @ 3:52 PM
Hi, it’s not actually a problem with the plugin but with that particular package. A solution is to change your nuget folder to c:\nuget to stay within the path limit.
25 August 2022 @ 7:28 PM
Hi! Nice job! Is possible to use this plugin with .NET MAUI Blazor App?
25 August 2022 @ 7:00 PM
Thank you for making using admob easy in net mau I was using it with xamarin.
I get the following error when i add banner in xaml:
Microsoft.Maui.Platform.HandlerNotFoundException: ‘Handler not found for view Plugin.MauiMTAdmob.Controls.MTAdView.
9 August 2022 @ 2:55 PM
I have always had a question about how to add advertisements to windows applications, so I would like to know if your work can support the windows11 platform in the future?
9 August 2022 @ 3:45 PM
Hi John. Currently Windows is not supported but it’s my intention to add support for it as soon as possible. Unfortunately admob is not supported on Windows so I need to find something else to use. Any suggestion would be appreciated!
7 August 2022 @ 6:10 PM
Severity Code Description Project File Line Suppression State
Error Could not find a part of the path ‘C:\Users\DAX.nuget\packages\xamarin.firebase.ios.core\8.10.0.1\lib\net6.0-ios15.4\Firebase.Core.resources\GoogleUtilitiesComponents.xcframework\ios-arm64_i386_x86_64-simulator\GoogleUtilitiesComponents.framework\PrivateHeaders\GULCCComponentContainerInternal.h’.
3 August 2022 @ 6:43 PM
hello frind , thank you for great blugin , i was using it in xamarin forms and it is very easy to use.
I am trying to use this for maui but I get an errors while instaling
there is a problem with xamarin.firebase.ios pakeges .
I am waiting for solving this problem , thanks again..
3 August 2022 @ 7:23 PM
Hello. What error do you see? Usually the error with firebase is because the path of that nuget package is too long. Try to reduce it. Anyway if you add more details I can try to identify your issue